Sending Mandrill email notifications in Laravel
Mandrill, a transactional email platform built by Mailchimp. We’ve been using it at Dubicars.com for few years now to deliver all sorts of emails to our users. Only recently we started using the package that’s being featured in this article, which is working smoothly only better with Laravel notifications.
composer require salamwaddah/laravel-mandrill-driver
If you want to send a Mandrill email as easy as the following the example using Laravel then continue reading because this package is for you.
use SalamWaddah\Mandrill\MandrillMessage;
public function toMandrill($notifiable)
{
return (new MandrillMessage())
->addTo($notifiable->email)
->subject('Purchase successful')
->view('template-name-on-mandrill', [
'user' => $notifiable,
'invoice_link' => 'https://example.com/download/invoice.pdf',
]);
}
Prerequisites
PHP 7 or higher
Laravel 5.4 or higher (Laravel 5, 6, 7)
Mandrill API key
Getting API key from Mandrill
Log into your Mandrill account and head to the settings tab to generate a new key. Click the button + New API Key to get the following dialog, and give your key a description, example “Staging test key”, in my case I named it “My Test Key”.
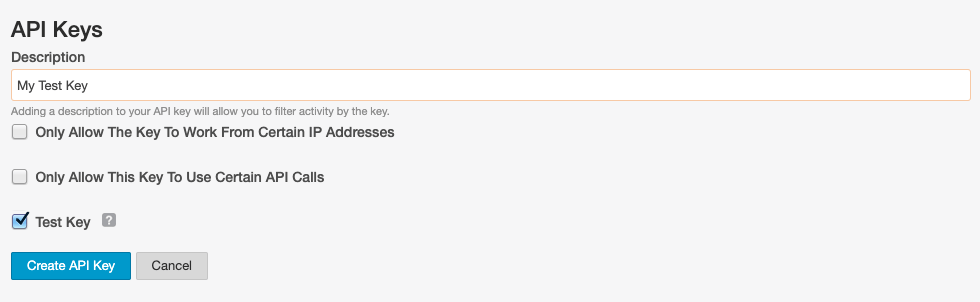
Don’t forget to enable “Test Key” if you don’t want Mandrill to send an actual email while testing,
You can use a test key to experiment with Mandrill’s API. No mail is actually sent, but webhooks trigger normally and you can generate synthetic bounces and complaints without impacting your reputation.
Now that you have an API key, copy it for later use, it will be listed like the following.

Installing and setting up the package
Using your terminal go to your Laravel project directory to install with composer using the command
composer require salamwaddah/laravel-mandrill-driver
Then in your .env file add the testing API key you copied earlier from Mandrill
MANDRILL_SECRET=YOUR_MANDRILL_API_KEY
Now, to mail.php file in your config directory, if the file doesn’t exist then create it, but it should be there as it’s a default Laravel file. Add the snippet:
'from' => [
'address' => '[email protected]',
'name' => "From Name"
],
'mandrill' => [
'key' => env('MANDRILL_SECRET')
]
That’s it, you’re all set to use the Mandrill notifications package for Laravel, onto the usage now.
Usage
Let’s first create a fresh Laravel notification class using the command
php artisan make:notification PurchaseSuccessful
By default, the new class will be created in the namespace App\Notifications so navigate there and have a look at the boilerplate and let’s start modifying it.
In the notification class you just created import the required classes for later use
use SalamWaddah\Mandrill\MandrillChannel;
use SalamWaddah\Mandrill\MandrillMessage;
Then let’s modify the via method, just Add MandrillChannel::class
. It’s up to you to remove the default 'mail' channel or keep it, but you probably won’t need it now that you’re gonna use Mandrill.
public function via($notifiable)
{
return ['mail', MandrillChannel::class];
}
Time to create toMandrill
method which will send your email content to mandrill.
The only thing you need from Mandrill in here is the template name
public function toMandrill($notifiable)
{
return (new MandrillMessage())
->addTo($notifiable->email)
->subject('Purchase successful')
->view('template-name-on-mandrill', [
'user' => $notifiable,
'invoice_link' => 'https://example.com/download/invoice.pdf',
]);
}
Notice here the view methods accepts 2 params, the first one is your mandrill template name, the second param is an array of whatever content you need to send to Mandrill. In my example I am sending in the content the user as array and invoice_link
as a string of a downloadable file.
If you need to pass additional data, let’s say an order object, you can do so in the notification constructor then pass the order to toMandrill
method like so;
protected $order;
public function __construct(Order $order)
{
$this->order = $order;
}
Sending the notification to Mandrill
Now that your notification class has a constructor (optional), and via, toMandrill methods let’s notify the user via mandrill.
$user->notify(new PurchaseSuccessful($order));
That’s it.
The $user
is an instance of your user in database, notify your user with a new PurchaseSuccessful
class and pass any data your constructor accepts.
Mandrill handlebars
The request is now sent to mandrill in handlebars syntax, and you can use it in your templates like this.
Hey {{user.name}}, you have successfully purchased, please download your invoice from {{invoice_link}}.
Summary
When your Laravel project is setup and your User
class is notifiable, easily use this package and use it like any other notification channel.
For advanced usage and list of available methods have a look at the documentation on Github, my future plan is to add tests, feel free to contribute.